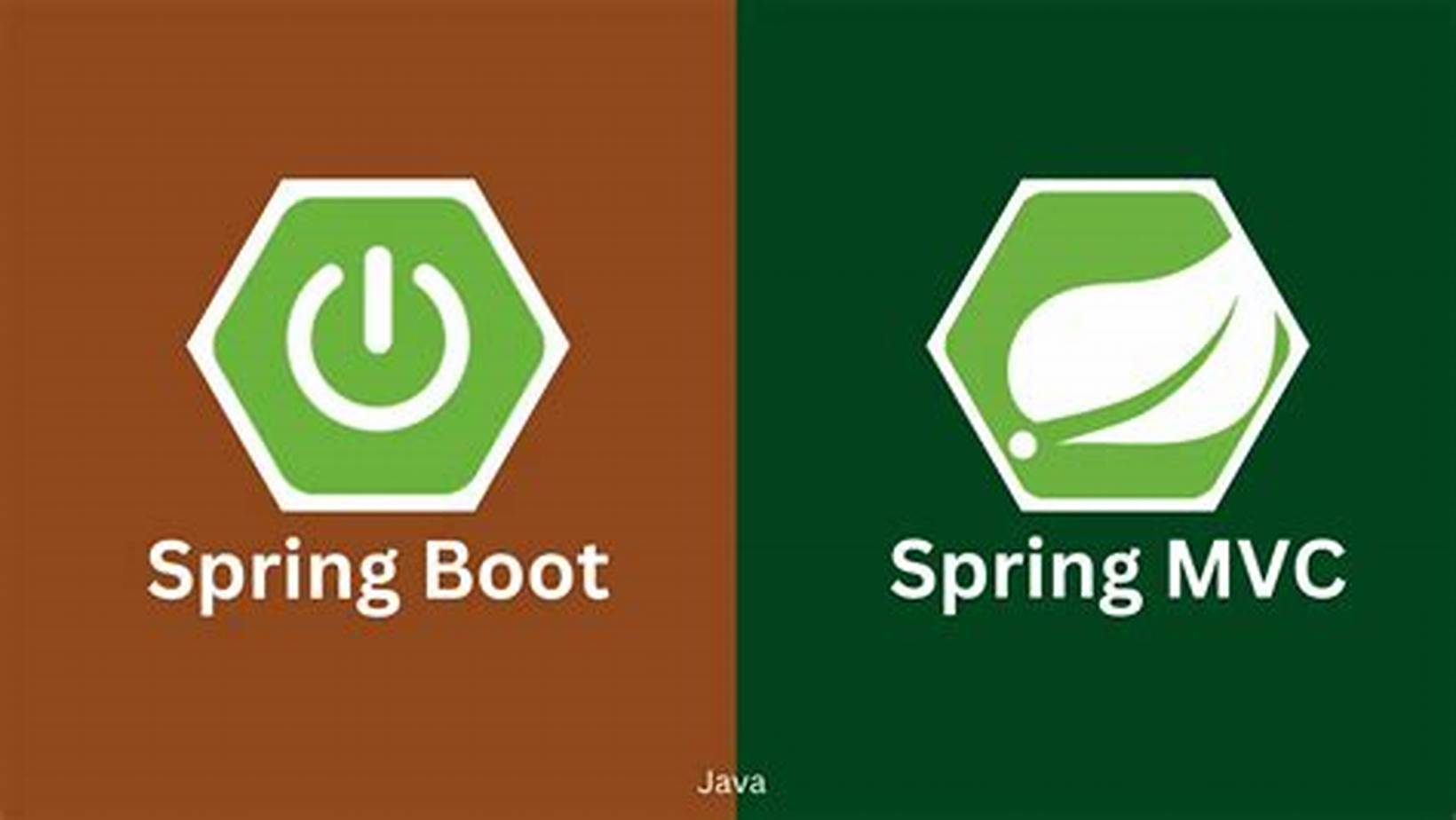
There are a two stable, mature stacks for building web applications in the Java ecosystem, and considering the popularity and strong adoption, the Spring Framework is certainly the primary solution.
Spring offers a quite powerful way to build a web app, with support for dependency injection, transaction management, polyglot persistence, application security, first-hand REST API support, an MVC framework and a lot more.
Traditionally, Spring applications have always required significant configuration and, for that reason, can sometimes build up a lot of complexity during development. That’s where Spring Boot comes in.
The Spring Boot aims to make building web application with Spring much faster and easier.
Let’s have a look at some of the important features in Boot:
- starter modules for simplifying dependency configuration.
- auto-configuration whenever possible.
- embedded, built-in Tomcat, Jetty or Undertow.
- stand-alone Spring applications.
- production-ready features such as metrics, health checks, and externalized configuration.
- no requirement for XML configuration.
Spring Boot Starters
To create a Spring Boot application, you first need to configure the spring-boot-starter-parent artifact in the parent section of the pom.xml:
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.5.3.RELEASE</version>
<relativePath /><!-- lookup parent from repository -->
</parent>
This way, you only need to specify the dependency version once for the parent. The value is then used to determine versions for most other dependencies — such as Spring Boot starters, Spring projects or common third-party libraries.
The advantage of this approach is that it eliminates potential errors related to incompatible library versions. When you need to update the Boot version, you only need to change a single, central version, and everything else gets implicitly updated.
A good starting point is creating a basic web application. To get started, you can simply add the web starter to your pom.xml:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
If you want to enable Spring Data JPA for database access, you can add the JPA starter:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
The Entry Point in a Boot Application
The entry point for a Spring Boot application is the main class annotated with @SpringBootApplication:
@SpringBootApplication
public class Application {
public static void main(String[] args){
SpringApplication.run(Application.class, args);
}
}
This is all we need to have a running Boot application.
The shortcut @SpringBootApplication annotation is equivalent to using @Configuration, @EnableAutoConfiguration, and @ComponentScan and will pick up all config classes in or bellow the package where the class is defined.
Embedded Web Server
Spring Boot launches an embedded web server when you run your application.
If you use a Maven build, this will create a JAR that contains all the dependencies and the web server. This way, you can run the application by using only the JAR file, without the need for any extra setup or web server configuration.
By default, Spring Boot uses an embedded Apache Tomcat 7 server. You can change the version by specifying the tomcat.version property in your pom.xml:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<exclusions>
<exclusion>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
</exclusion>
</exclusions>
</dependency>
Not surprisingly, the other supported embedded servers are Jetty and Undertow. To use either of these, you first need to exclude the Tomcat starter:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<exclusions>
<exclusion>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
</exclusion>
</exclusions>
</dependency>
Then, add the Jetty or the Undertow starters:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jetty</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-undertow</artifactId>
</dependency>
Spring Boot have many more advanced features which we will cover in next blog.
Thank You!!!!